13.6. Basic iterator operations¶
By design, iterators in C++ feel as if you are using pointers. Most iterators support the same operations as pointers.
Operation |
Result |
---|---|
|
|
|
negation of above |
|
refers to the element pointed to by |
|
writes |
|
reads from the element pointed to by |
|
increment the iterator, making it point to the next
element in the container or to |
13.6.1. Iterator categories¶
Different containers need different capabilities from their iterators.
Instead of being defined by specific types, each category of iterator is defined by the operations that can be performed on it. This definition means that any type that supports the necessary operations can be used as an iterator — for example, a pointer supports all of the operations required by Random Access Iterator, so a pointer can be used anywhere a Random Access Iterator is expected.
All of the iterator categories (except Output Iterator) can be organized into a hierarchy, where more powerful iterator categories Random Access Iterator) support the operations of less powerful categories (e.g. Input Iterator). If an iterator falls into one of these categories and also satisfies the requirements of Output Iterator, then it is called a mutable iterator and supports both input and output. Non-mutable iterators are called constant iterators.
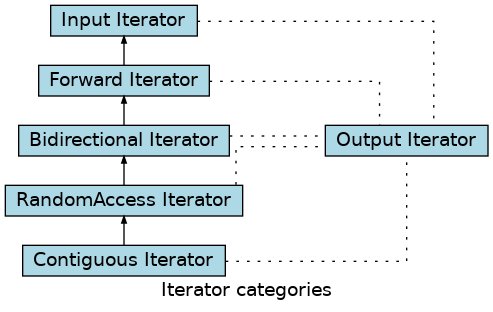
- Input Iterator
Read elements and increments using
operator++
, without multiple passes. Classes like basic_istream provide this iterator.- Forward Iterator
InputIterator, plus increment using
operator++
, with multiple passes. The forward_list container provides this iterator.- Bidirectional Iterator
ForwardIterator, plus decrement using
operator--
Containers like list, map, and set provide this iterator.- Random Access Iterator
BidirectionalIterator, plus access using
operator[]
Before C++17, containers like vector, array, and string provided this iterator. It is still used for unordered collections like unordered_map and unordered_set- Contiguous Iterator
RandomAccessIterator, plus the container makes a continuous storage guarantee. This category was added in C++17. Before C++17, iterators of containers like vector and array were often treated as a separate category. This category simply formalizes what was happening in practice.
- Output Iterator
More of a ‘sub category’ of all of the others. If the iterator allows writing to the element, it is also an OutputIterator An output iterator can only be dereferenced on the left-hand side of an expression:
vector<int> v = {1,2,3}; auto it = v.begin(); *it = 0;
More to Explore
Iterator Library at cppreference.com
C++ Named Requirements: Iterator