8.6. Pass by value¶
When you pass a structure as an argument, remember that the argument and
the parameter are not the same variable. Instead, there are two
variables (one in the caller and one in the callee) that have the same
value, at least initially. For example, when we call print_point
,
the stack diagram looks like this:
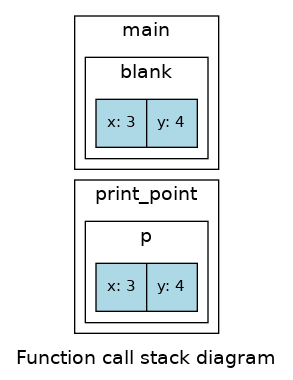
If print_point
happened to change one of the instance variables of
p
, it would have no effect on blank
. Of course, there is no
reason for print_point
to modify its parameter, so this isolation
between the two functions is appropriate.
This kind of parameter-passing is called “pass by value” because it is the value of the structure (or other type) that gets passed to the function.
Remember pass by value will always make a copy, leaving the original unchanged.
Take a look at the active code below.
Notice from the output of the code below how the
function add_two
changes the instance variables, but not on blank
itself.
- 2 4
- Take a look at exactly what is being output.
- 2 4 2
- Correct!
- 4 4 2
- Take a look at exactly what is being output.
- 2 4 4
- Remember the rules of pass by value.
Q-2: What will print?
int add_two(int x) {
cout << x << ' ';
x = x + 2;
cout << x << ' ';
return x;
}
int main() {
int num = 2;
add_two(num);
cout << num << '\n';
}
- 6.0, 8.0, 3.0, 4.0
- Correct!
- 6.0, 8.0, 6.0, 8.0
- Remember the rules of pass by value.
- 6.08.03.04.0
- Take a look at exactly what is being outputted.
- 6.08.06.08.0
- Take a look at exactly what is being outputted.
Q-3: What will print?
struct point {
double x;
double y;
};
void times_two (point p) {
cout << '(' << p.x * 2 << ", " << p.y * 2 << ')';
}
int main() {
point blank = { 3.0, 4.0 };
times_two (blank);
cout << ", " << blank << endl;
}