8.8. Rectangles¶
Now let’s say that we want to create a structure to represent a rectangle. The question is, what information do I have to provide in order to specify a rectangle? To keep things simple let’s assume that the rectangle will be oriented vertically or horizontally, never at an angle.
There are a few possibilities: I could specify the center of the rectangle (two coordinates) and its size (width and height), or I could specify one of the corners and the size, or I could specify two opposing corners.
The most common choice in existing programs is to specify the upper left
corner of the rectangle and the size. To do that in C++, we will define
a structure that contains a point
and two doubles.
struct rectangle {
point corner;
double width, height;
};
Notice that one structure can contain another. In fact, this sort of
thing is quite common. Of course, this means that in order to create a
rectangle
, we have to create a point
first:
point corner = { 0.0, 0.0 };
rectangle box = { corner, 100.0, 200.0 };
This code creates a new rectangle
structure and initializes the
instance variables. The figure shows the effect of this assignment.
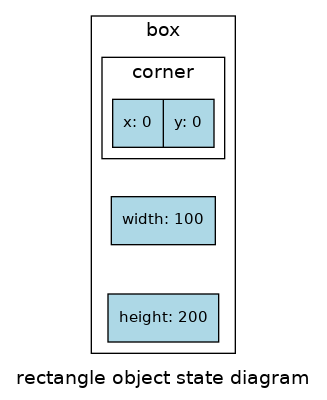
We can access the width
and height
in the usual way:
box.width += 50.0;
cout << box.height;
In order to access the instance variables of corner
, we can use a
temporary variable:
point temp = box.corner;
double x = temp.x;
Alternatively, we can compose the two statements:
double x = box.corner.x;
It makes the most sense to read this statement from right to left:
“Extract x
from the corner
of the box
, and assign it to the
local variable x
.”
While we are on the subject of composition, I should point out that you
can, in fact, create the point
and the rectangle
at the same
time:
rectangle box = { { 0.0, 0.0 }, 100.0, 200.0 };
The innermost curly braces are the coordinates of the corner point;
together they make up the first of the three values that go into the new
rectangle
.
This statement is an example of a nested structure.
The active code below uses the rectangle
structure. Feel free to
modify the code and experiment around!
- double x = corner.box.x;
- Try again.
- double x = box.corner.x;
- Correct!
- double x = corner.x;
- Try again.
- double x = box.x;
- Try again.
Q-2: How can you combine these two statements into one?
point temp = box.corner;
double x = temp.x;
def main() { point corner = { 0.0, 0.0 ); rectangle box = { ( 0.0, 0.0 ), 100.0, 200.0 } rectangle box = { { 0.0, 0.0 }, 100.0, 200.0 }; point corner = { 0.0, 0.0 }; rectangle box = { corner, 100.0, 200.0 }; }